Installation
Note: Addons require the latest bleeding edge version (nightly branch).
Composer
If your Addon exists on Packagist you can install it like any other package through the command line.
$ composer require acme/my-addon
Skip to Post-Installation
Local Development
We can leverage Composer repositories for installing a local package to speed up development efforts. This requires you have a local installation of FusionCMS.
Read more about Composer repositories here.
Below is a sample of an Addon including a local package.
{
"require": {
"acme/myaddon":"*"
// ...
},
"repositories": [
{
"type": "path",
"url": "../acme-myaddon",
"options": {
"symlink": true
}
}
]
}
Finally, run composer install
your local Addon package will be symlinked into FusionCMS.
Post-Installation
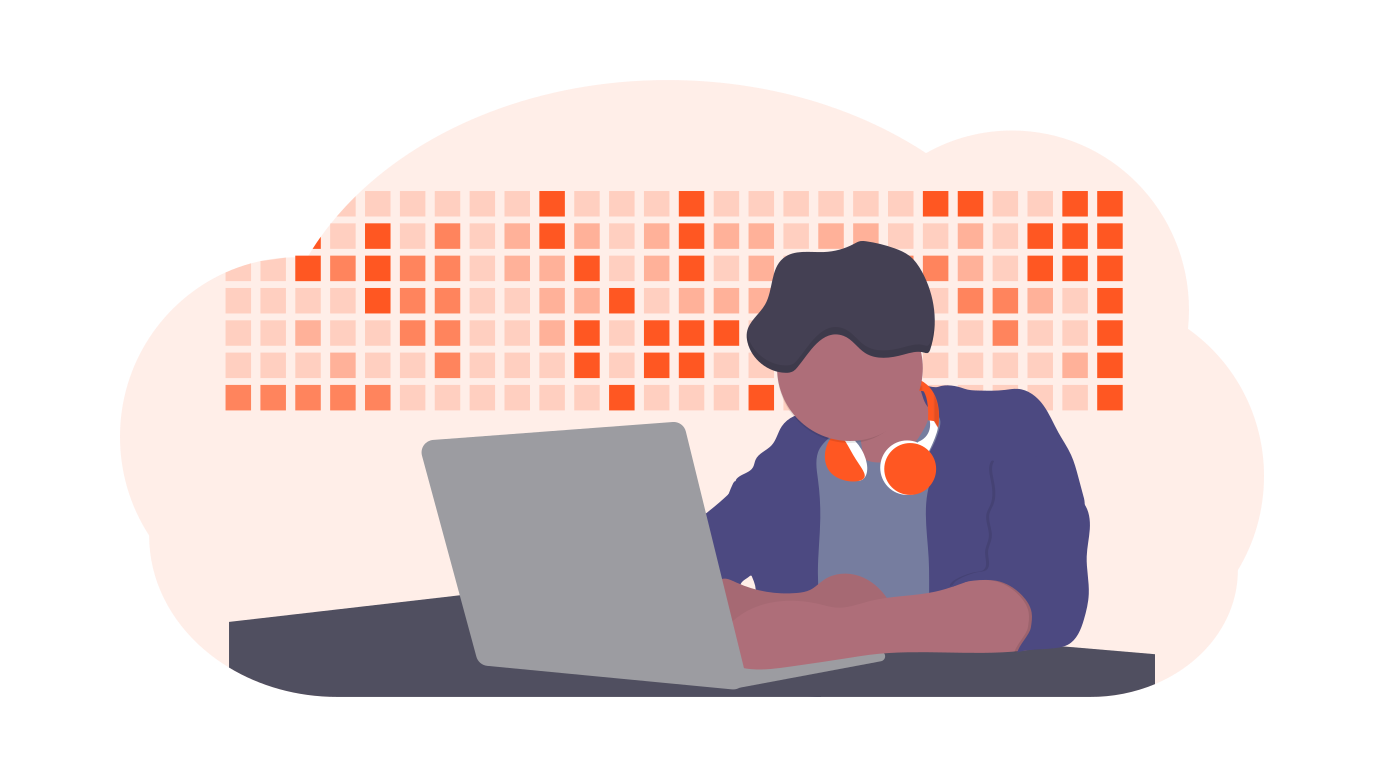
After composer installs your Addon, a couple additional steps happen:
- Laravel will clear it's
bootstrap/cache
(e.g. registered Service Providers and previously discovered Composer Packages). - Package Discovery using Illuminate\Foundation\PackageManifest. Read more on Laravel.
- Addon Discovery using
Illuminate\Foundation\PackageManifest
, however adapted to search for additional hints. See [Addon Discovery](#Addon Discovery) for more information. - FusionCMS Sync - Internal command, which re-discovers and registers stuff like permissions and settings. See [FusionCMS Sync](#FusionCMS Sync) for more information.
Addon Discovery
FusionCMS has it's own built-in registry for discovering new Addons packages. The registry is controlled by Fusion\Services\Addons\Manifest
and is responsible for reading all available packages and registering Addon's through a user-specified hint required in the composer.json
file:
// https://github.com/fusioncms/quotes-module/blob/master/composer.json
"extra": {
"fusioncms": {
"name": "Quotes"
},
"laravel": {
"providers": [
"Addon\\Quotes\\Providers\\QuotesServiceProvider"
]
}
}
FusionCMS will expect every Addon to have a composer.json
file with at least a extra.fusioncms
object. Discovered Addons are then cached in bootstrap/cache/addons.php
.
Manual Usage
Invoke manually through the command line:
$ php artisan addon:discover
FusionCMS Sync
FusionCMS provides a command to sync content from internal updates and discovered Addon packages. Below is a graphic to help visualize the processes happening behind the scenes.
Notice: This can be considered a destrictive command considering it will remove records, which have since been removed from schema.
Sync Addons
Runs Addon migrations (only once), and publishes any assets (none by default; can be registered through your Addon's Service Provider). See more information here.
Sync Resources
This will assure your Addon's resources are recognized by FusionCMS and your website. (i.e. The quotes-module will have a symlink named public/vendor/quotes
).
Sync Settings
Will register any settings included in your Addon. See full article for more information.
Sync Permissions
Will register any permissions included in your Addon. See full article for more information.
Sync Notifications
Will register any notifications included in your Addon. See full article for more information.
Manual Usage
Invoke manually through the command line:
$ php artisan fusion:sync
Manifest
Access the Addon Package Manifest through app('addons.manifest')
.
Note: This may turn into a facade at a later date.
Some conveinence methods are available:
$manifest = app('addons.manifest');
// Returns Illuminate\Support\Collection of Addons
$manifest->getAddons();
// Returns specific of Fusion\Services\Addons\Addon instance.
$manifest->getAddon('quotes');
// Returns boolean value for Addon's existance.
$manifest->hasAddon('quotes');
Addon
Requests for Addons through Manifest will wrap the Addon in a Fusion\Services\Addons\Addon
wrapper class.
Some conveinence methods are available:
$quotes = $manifest->getAddon('quotes');
// Returns slug representation
$quotes->getSlug();
// Returns tag to use for publishing
$quotes->getPublishTag()
// Returns fully qualified path to Addon
$quotes->getPath($path = '');
Uninstall
You can remove your Addon like so:
$ composer remove acme/my-addon
Behind the scenes, FusionCMS will handle triggering the Addon to uninstall itself (e.g. rollback migrations, and unpublished any assets), and afterwards composer refreshes itself and a couple other housekeeping processes are ran.
The important thing is it's best to remove your package through composer so these inner processes can run allowing FusionCMS to stay clean.
Testing
A mock
method is provided in order to skip the build
call, which can infect the main project.
// tests/src/Feature/Addons/AddonTest.php
use Illuminate\Support\Facades\Artisan;
// Mock w/ Addon..
$manifest = app('addons.manifest');
$manifest->mock($this->getBasePath('../addons/acme/myaddon'));
// Re-sync..
Artisan::call('fusion:sync');
Now you can run tests as if your Addon is part of FusionCMS!