What is a theme?
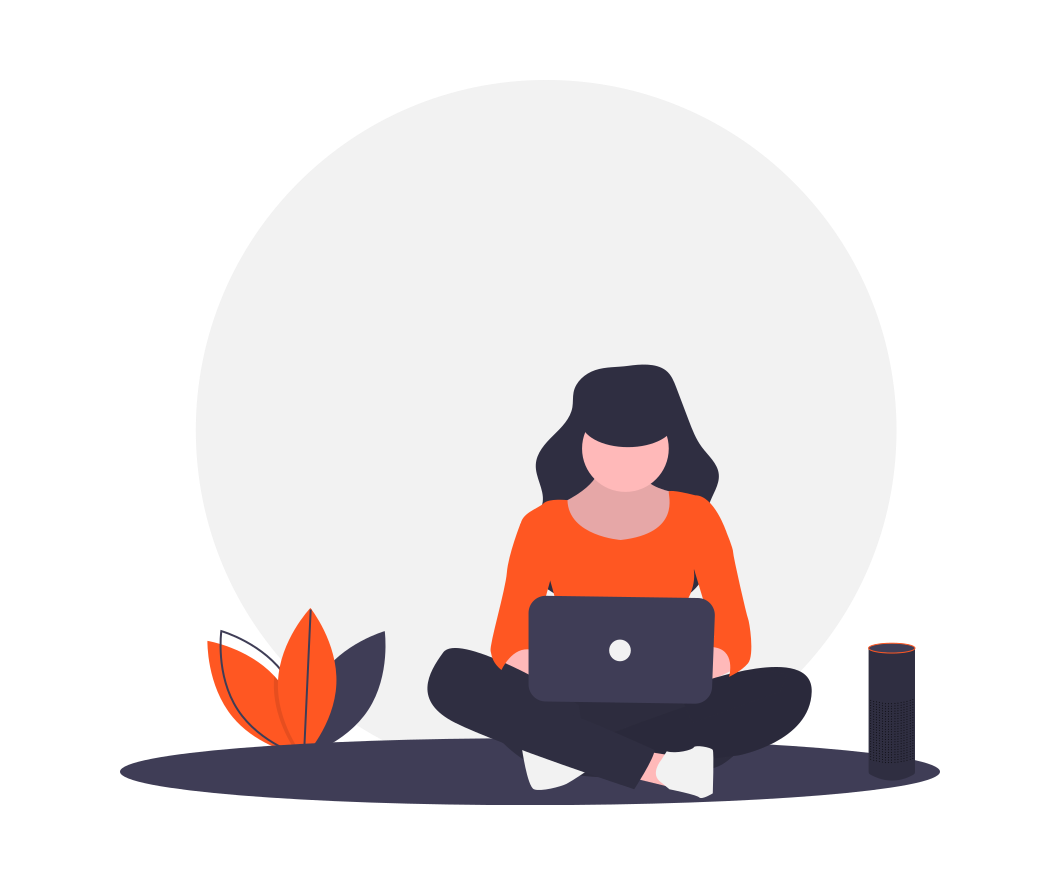
A FusionCMS theme is a collection of files & resources that dictate the frontend design & functionality of your website. Themes drive the way in which users will interact and experience websites built on FusionCMS and are built around your unique content structure. Themes are completely file-based, making them easy to work with and version control under Git or any other VCS platform of your choosing. A few features of themes:
- Powered by Laravel Blade - FusionCMS themes utilize the Blade templating engine provided by Laravel to power and drive the dynamic templating functionality of your view files.
- First-Class Citizens - We developed the theming system to elevate themes as first-class citizens within FusionCMS. They have access to the core API, meaning native functionality and routes may be baked into the front end or even extend functionality of the CMS backend.
- Developer Focused - Theme structure requirements are minimal, allowing you as the developer complete control of the frontend build process and framework.
- Customizable - Themes may have configurable settings to easily customize their look & feel on the fly through the FusionCMS theme customizer.
- Quick Scaffolding - Themes can be paired with content structure blueprints to easily scaffold an entire site build at the click of a button.
In this documentation, you will find everything you need to know in order to create beautiful and interactive themes based on any design.
Structure of a Theme
The basic structure required for themes is as follows:
site
└── themes
└── YourTheme
├── public
└── resources
└── views
In addition, every theme should contain a theme.json
file and preview.png
file at it's root. Edit the theme.json
to change basic information about a theme or to add advanced configurations, like customizable theme settings.
public
The public folder contains your finalized CSS files, JS files and other assets required by your theme. Other assets would include things like images and font files. These files need to reside within the public directory in order to be accessible by the web. The following subdirectory structure would be suggested for your public folder, but you may arrange things as you like here:
public
├── css
├── fonts
├── images
└── js
resources
The resources folder contain your views
folder for blade layouts, partials and templates. This folder may also contain any other source files required by your theme. If you're using pre-processors for your CSS or JS, this would be a good place to put your pre-compiled source files. Here is an example of a subdirectory structure for resource files:
resources
├── scss
├── js
└── views
views
The views folder contains all your blade template files. Create as many subfolders necessary to organize your templates. An index.blade.php
file should always exist directly in your views folder, this page will be auto-loaded as the homepage.
Note: It's highly recommended to create subfolders within views for the three common view file types you'll use in building your theme; layouts, partials and templates.
How do I create a new theme?
Option 1: make:theme
command
The quickest way to get going with a new theme is Fusion's Artisan make:theme
command. SSH into your local server at the root of your project and run the command below, replacing {MyTheme}
with the desired name of your new theme and follow all prompted instructions. Please note that all theme names should be in PascalCase format using letters and numbers only.
php artisan make:theme {MyTheme}
This will generate a basic theme in your site/themes
folder and auto-fill the theme.json
file with your input information. You may use this as a starting point for your own theme development. This auto-generated theme comes with Laravel Mix for JS & SCSS compilation via Webpack using the folder structure outlined above.
If this auto-generated theme does not align well with your build process and you'd prefer to create your own boilerplate for new FusionCMS themes, the make:theme
command provides a way to do so with a template
flag, replacing {Starter}
with the name of your boilerplate template:
php artisan make:theme {MyTheme} --template={Starter}
It is highly suggested to use the generated theme as reference for any necessary layout tags, even if you'd like to put a different build process in place. Please see documentation on "Building Boilerplate Templates" (coming soon..) for more information on creating your own.
Option 2: Manually Configure
Of course, you have the option to forgo the artisan command completely and manually copy an existing theme into a new folder under site/themes
. Please keep in mind that PascalCase format naming conventions still apply to your new theme folder name as well as the namespace
value in the theme.json
file. Both the folder name and namepace
values should match. The namespace may need to be replaced in other files throughout the theme as well. Please do a find and replace of the old name space to quickly swap out for the new one.
Example theme.json
:
{
"name": "My Theme",
"namespace": "MyTheme",
"description": "This is my own very special theme built by me!",
"author": "John Doe",
"version": "1.0.0"
}
Accessing theme files
All theme assets like CSS, JS and image files should reside in the theme's public
folder. The public files of the active theme will generate an automatic symlink to FusionCMS's public folder. For this reason, when referencing these files from your theme you can access them via a main /theme
path followed by the subdirectory structure you have set up.
Assuming the following structure:
public
├── css
| └── theme.css
├── images
| └── logo.png
└── js
└── theme.js
You can access each of these files via the /theme
path in your HTML markup.
// CSS
<link rel="stylesheet" href="/theme/css/theme.css" media="screen">
// JS
<script href="/theme/js/theme.js"></script>
// Image
<img src="/theme/images/logo.png" alt="logo">
Files and assets can be referenced in the same way in CSS.
background: url('/theme/images/galaxy.png');
@font-face {
font-famly: 'Roboto';
src: url('/theme/fonts/Roboto.eot');
...
}
Getting started with helpers
FusionCMS includes a variety of global "helper" PHP functions. Here we've listed a few important ones to get started with that will make theme development a little easier. You can find a complete list of our available functions in the Helpers document.
setting
There will be instances where you need to access a CMS setting value to make determinations about things in your theme. The setting
function will reference all items under Settings in the CMS by their type & field handle, formatted like setting('type.handle')
.
An example would be accessing the website name for a page title. You can find this value under Settings > System in the CMS. So in this case the type would be system
. The name of the field is "Website Title" so the handle would be formatted as website_title
.
<title>{{ setting('system.website_title') }} - {{ setting('system.website_slogan') }}</title>
theme
The theme
function allows you to access data from the theme.json
file. This includes things like the name, author, description or version of your theme.
The {{ theme('name') }} theme was made by {{ theme('author') }} and is on version {{ theme('version') }}.
You can build off of this file and add your own data about your theme.
For example, if your theme uses images throughout the design it can be really helpful to assign these images in the theme.json
and call them in your views using the theme
function.
"images": {
"loading": "/theme/images/loading.svg",
"logo": "/theme/images/logo.svg"
}
If you ever update, rename or move these images, you will only have to change the values in the theme.json
instead of tracking down all the instances where you've used them in your views.
<img class="lazyLoad" src="{{ theme('images.loading') }}" data-src="{{ theme('images.logo') }}" alt="Logo">
The theme.json
file is also where theme customization settings will live and can be accessed via theme
function, but in a slightly different manner. Please check out Customization Docs for more complete information on creating and using theme customization settings.
theme_mix
Because FusionCMS is tied so closely with the Laravel ecosystem, we use and recommend Laravel Mix for bundling and compiling JS/SCSS/LESS.
Many developers suffix their compiled assets with a timestamp or unique token to force browsers to load the fresh assets instead of serving stale copies of the code. One of the benefits of using Laravel Mix is automatic file versioning with hash values. Every time a new version of a CSS or JS file is compiled, a hash value can be automatically appended to the end of the file allowing for more convenient cache busting.
<script src="/theme/js/theme.js?id=xxxxxxxx"></script>
After generating the versioned file, you won't know the exact file name. So, you should use the theme_mix
within your views to load the appropriately hashed asset. This will automatically determine the current name of the hashed file for you:
<script src="{{ theme_mix('js/theme.js') }}></script>
Please checkout the Laravel Mix documentation or Mix on github for more information on how to set up and use Mix.
FAQs
How do I set an active theme?
Navigate to the Configure > Theme page in the CMS. In the upper right corner of this page, click the "Browse" button. This will list all the themes you currently have available to use. Locate the theme you wish to use on your site and click "Set as active".
How do I install a theme?
If you'd like to install an existing theme, simply drop the theme folder into your site/themes
folder. It will now show up as an option when you browse your list of installed themes.
Can I switch between themes in the CMS?
Each site build is unique, featuring a customized content structure configured through the CMS. So while it's possible to have multiple themes installed and switch between them, the content structure anticipated by your new theme might not match the content structure of your old theme. In this case, either your content structure will need to be re-configured or your new theme will need to be updated to account for the new structure.