Getting Started
You are welcome to create your Addon from scratch. If you do, reference the Starting Template section for a minimal required setup. Otherwise, you can generate a template through the command line.
Generate Template
First you'll need to download FusionCMS' own command line tool: fusioncms/cli
$ composer -g require fusioncms/cli
Now generate a fresh template with the following command:
$ fusion make:addon acme-myaddon
Your Addon will be generated within a newly created acme-myaddon
folder with namespace Acme\Myaddon
.
Starting Template
-- acme-myaddon/
| -- config/
| -- myaddon.php
| -- public/
| -- resources/
| -- views/
| -- lang/
| -- routes/
| -- api.php
| -- web.php
| -- src/
| -- Providers/
| -- MyaddonServiceProvider.php
| -- composer.json
Extending
Your Addon extends the functionality of FusionCMS through the following directories:
Folder | Description |
---|---|
config/ |
Laravel configurations. [See Configurations] |
database/migrations/ |
Database migration files. [See Migrations] |
database/factories/ |
Model factory files. [See Factories] |
notification/ |
Notification files. [See Notifications] |
permissions/ |
Permission configurations. |
settings/ |
Setting configurations. |
src/Console |
Console commands. [See Artisan] |
src/Fieldtypes |
FusionCMS Fieldtypes. |
Installation
Note: Addons require the latest bleeding edge version (nightly branch).
Composer
If your Addon exists on Packagist you can install it like any other package through the command line.
$ composer require acme/my-addon
Afterwards, FusionCMS will register your Addon and run any setup commands.
Local Development
We can leverage Composer repositories for installing a local package to speed up development efforts. This requires you have a local installation of FusionCMS.
Read more about Composer repositories here.
Below is a sample of an Addon including a local package.
{
"require": {
"acme/myaddon":"*"
// ...
},
"repositories": [
{
"type": "path",
"url": "../acme-myaddon",
"options": {
"symlink": true
}
}
]
}
Finally, run composer install
your local Addon package will be symlinked into FusionCMS.
Addon Configurations
Getting Started
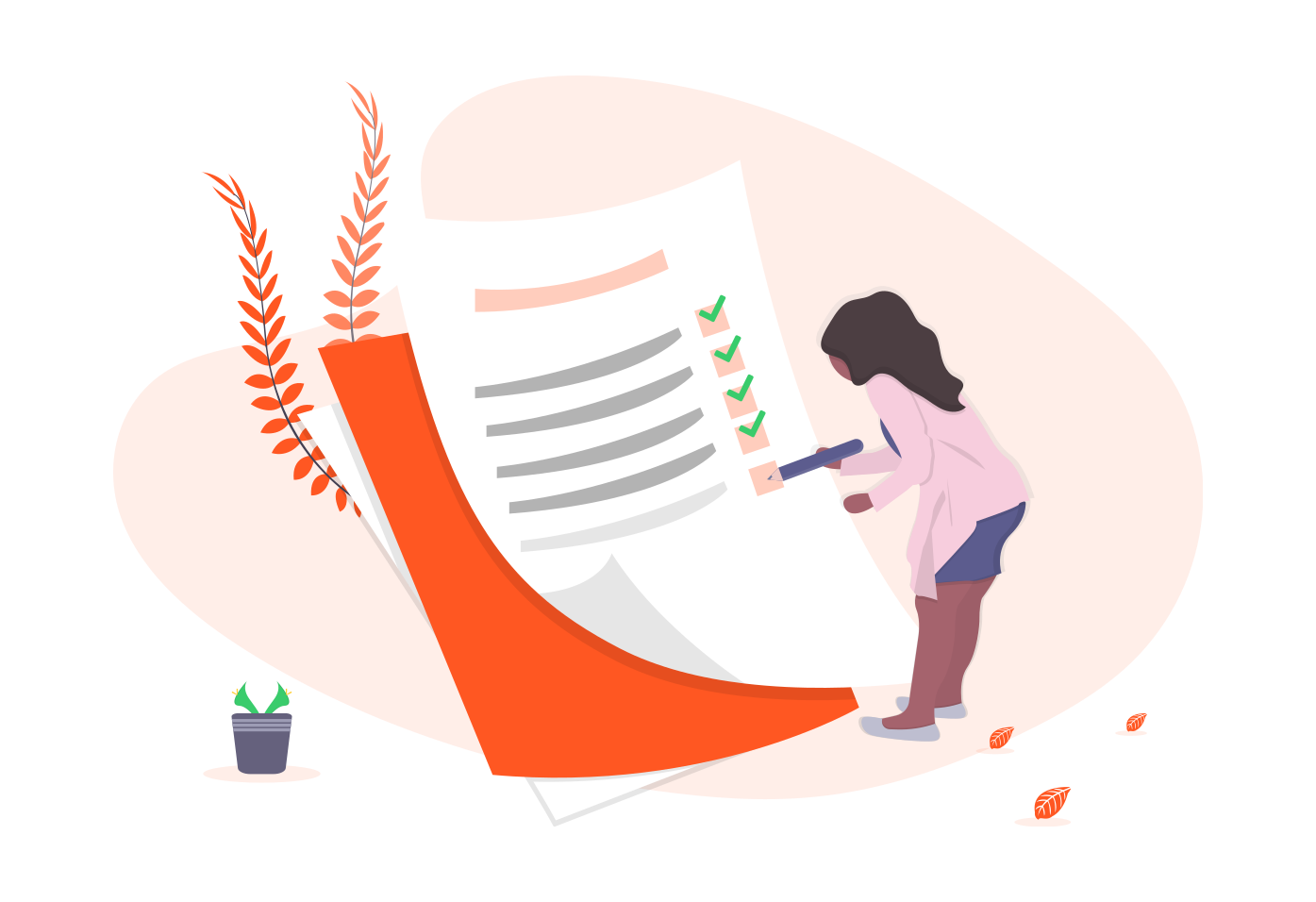
The main entry for every Addon is the Service Provider within src/Providers
. This is where all Addon configurations will reside. There is no naming convention, however it's required that you include all Service Providers you want loaded in the extra.laravel.providers
namespace of your composer.json
file.
{
"extra": {
"laravel": {
"providers": [
"Acme\\MyAddon\\Providers\\AcmeServiceProvider"
]
}
}
}
See fusioncms/quotes-module for full example Addon.
On every page request, the Service Provider's register
and boot
methods will be called along with all other Service Providers. It is recommended at least one Service Provider extends from Fusion\Providers\AddonServiceProvider
to let FusionCMS automate the process:
<?php
namespace Addon\Quotes\Providers;
use Fusion\Providers\AddonServiceProvider;
class QuotesServiceProvider extends AddonServiceProvider
{
//...
}
You are welcome to control how your Addon loads it's necessary configurations. For more information see Writing Service Providers.
Commands
Extend FusionCMS' command line tools through commands.
Registration
Register your commands through your Addon's Service Provider by overriding the $commands
property.
use Acme\MyAddon\Console\BillTedCommand;
protected $commands = [
BillTedCommand::class,
];
Class
You will be responsible for creating the commnd within your Addon folder structure under src/Console
. The following is a sample command.
<?php
namespace Acme\MyAddon\Console;
use Illuminate\Console\Command;
class AdventureCommand extends Command
{
/**
* The name and signature of the console command.
*
* @var string
*/
protected $signature = 'bill:ted';
/**
* The console command description.
*
* @var string
*/
protected $description = 'Take a bogus journey.';
/**
* Execute the console command.
*
* @return mixed
*/
public function handle()
{
return $this->info('No Way!!');
}
}
Usage
You can run your command through FusionCMS:
$ php artisan bill:ted
> No Way!!
Configurations
Additional configurations can be added to config/{slug}.php
file. The file must be named after the {slug}
of your Addon.
Class
Configurations are stored as a multi-dimensional array. Read the Laravel docs for more information on how to access and set configurations.
<?php
return [
'allow_comments' => true
];
Usage
You can call your configurations using Laravel's helper method like so.
config('acme.allow_comments'); // => true
Database
Migrations
Files added to database/migrations
will automatically be registered as migration files.
Factories
Files added to database/factories
will automatically be registered as model factories files.
Events
Event Listeners
Register event listeners by overriding the $listen
property in your Service Provider.
use Acme\MyAddon\Events\SomethingHappend;
use Acme\MyAddon\Listeners\HandleSomething;
protected $listen = [
SomethingHappend::class => [
HandleSomething::class,
],
];
Event Subscribers
Register event subscribers by overriding the $subscribe
property in your Service Provider.
use Acme\MyAddon\Listeners\AddonSubscriber;
protected subscribe = [
AddonSubscriber::class
];
Routing
Register your routes in the provided routes/web.php
& routes/api.php
files. Visit Laravel's routing documentation page for more information on how to configure routes.
Control Panel Routing
In order to include links in the sidebar of the Control Panel override the $navigation
property. This will create the link in the Control Panel's sidebar.
<?php
namespace Acme\MyAddon\Providers;
use Fusion\Providers\AddonServiceProvider;
class AcmeServiceProvider extends AddonServiceProvider
{
/**
* FusionCMS CP navigation.
*
* @var array
*/
protected $navigation = [
'title' => 'Acme',
'to' => '/acme',
'icon' => 'acme'
];
}
Route Model Binding
FusionCMS has the following route model bindings: blueprint
, directory
, fieldset
file
, navigation
, taxonomy
, user
. Implicit binding should resolve your model, however if for explicit bindings, include them into your boot method.
<?php
namespace Addon\MyAddon\Providers;
use Acme\MyAddon\Models\Acme;
use Fusion\Providers\AddonServiceProvider;
use Illuminate\Support\Facades\Route;
class AcmeServiceProvider extends AddonServiceProvider
{
public function boot()
{
parent::boot();
Route::model('acme', Acme::class);
}
}
Middleware
Register middleware for route groups in your Addon's Service Provider.
use Acme\Myaddon\Http\Middleware\MyAPIMiddleware;
use Acme\Myaddon\Http\Middleware\MyWebMiddleware;
protected $middlewareGroups = [
'web' => [
MyWebMiddleware::class
],
'api' => [
MyAPIMiddleware::class
]
];
Resources
Views
Any files within resources/views
will automatically be registered as View files.
Translations
Any files within resources/lang
will automatically be registered as Translations files.
Task Scheduling
Include any tasks to be scheduled within the boot
method of your Addon's service provider.
<?php
namespace Addon\MyAddon\Providers;
use Fusion\Providers\AddonServiceProvider;
use Illuminate\Console\Scheduling\Schedule;
class AcmeServiceProvider extends AddonServiceProvider
{
public function boot()
{
parent::boot();
$this->callAfterResolving(Schedule::class, function (Schedule $schedule) {
$schedule->command('acme:inspire')->daily();
});
}
}
Extending
Fieldtypes
FusionCMS already provides a vast collection of Fieldtypes, however you may wish to add another. Register your Fieldtypes by overriding the $fieldtypes
property in the Service Provider.
<?php
namespace Addon\MyAddon\Providers;
use Acme\MyAddon\Fieldtypes\MyFieldType;
use Fusion\Providers\AddonServiceProvider;
class AcmeServiceProvider extends AddonServiceProvider
{
protected $fieldtypes = [
MyFieldType::class,
];
// ...
}
After your Fiedltype has been registered there's still the JS portion to complete. See the full article here.
Settings
You can introduce additional settings to FusionCMS's Control Panel. These settings can be then used within FusionCMS to control certain aspects of the system.
// Example
return [
'name' => 'WarpSpeed',
'description' => 'Settings for the WarpSpeed Addon.',
'group' => 'Addons',
'icon' => 'starship',
'settings' => [
'General' => [
[
'name' => 'Top Speed',
'handle' => 'warpspeed_top_speed',
'description' => 'Maximum speed setting.'
'type' => 'number',
'default' => 10
],
]
]
];
For more information see the full article.
Merged in through
php artisan fusion:sync
.
Notifications
Register notifications.
// Example
return [
'WarpSpeed' => [
'MaximumWarp' => WarpSpeed\Notifications\MaximumWarp::class,
]
];
For more information see the full article.
Merged in through
php artisan fusion:sync
.
Permissions
// Example
return [
'warp' => ['viewAny', 'view', 'create', 'update', 'delete'],
];
See permissions page for more information.
Merged in through
php artisan fusion:sync
.
Front-End
JavaScript and CSS Resources
Your Addon will be responsible for compiling Javascript and CSS assets into the public/
folder. Register your front-end assets in your Addon's Service Provider by overriding $assets
.
protected $assets = [
'/vendor/{slug}/js/{slug}.js',
'/vendor/{slug}/css/{slug}.css'
];
*
slug
= slugified version of your Addon's name.
CLI Commands
The following Artisan commands can be run through the command line.
Command | Description |
---|---|
addon:discover |
Discover and register all addons. |
addon:list |
List all registered addons. |